Once upon a time, a student came to Master Coffee and complained about Javascript being “crazy and stupid”. Yes, how is it that 1 + 1 = 11
? Well, this is a common pitfall and misunderstanding among beginners. Let Master Coffee explain and show you the proper ways. Let’s go.
CODE DOWNLOAD
I have released this under the MIT license, feel free to use it in your own project – Personal or commercial. Some form of credits will be nice though. 🙂
VIDEO TUTORIAL
1) CONCAT VS ADD
<!-- (PART A) DEMO FORM -->
<form onsubmit="return add()">
<input type="number" id="numA" value="1">
<input type="number" id="numB" value="1">
<input type="submit" value="Add">
</form>
<!-- (PART B) ADD "NUMBERS" -->
<script>
function add () {
// (B1) BOTH NUM A & NUM B ARE STRINGS!
let numA = document.getElementById("numA").value;
let numB = document.getElementById("numB").value;
console.log(typeof numA);
console.log(typeof numB);
// (B2) THIS IS CONCAT, NOT SUM
let sum = numA + numB;
console.log(sum);
return false;
}
</script>
First, let us debunk the mystery of 1 + 1 = 11
.
- (A) Just a usual HTML form, with 2 number fields.
- (B1) Take note, the values obtained from the fields are of string datatype.
- (B2) So what we are doing here is not adding 2 numbers, but concatenating 2 strings –
"l" + "1" = "11"
.
2) PARSE STRING TO NUMBER
<!-- (PART A) DEMO FORM -->
<form onsubmit="return add()">
<input type="number" id="numA" value="1">
<input type="number" id="numB" value="1.1">
<input type="number" id="numC" value="1.2">
<input type="submit" value="Add">
</form>
<!-- (PART B) ADD NUMBERS -->
<script>
function add () {
// (A1) PARSE AS NUMBERS THEN ADD
let numA = parseInt(document.getElementById("numA").value);
let numB = parseFloat(document.getElementById("numB").value);
let numC = Number(document.getElementById("numC").value);
sum = numA + numB + numC;
console.log(typeof numA);
console.log(typeof numB);
console.log(typeof numC);
console.log(sum);
// (A2) SHORT HAND
sum =
+document.getElementById("numA").value +
+document.getElementById("numB").value +
+document.getElementById("numC").value;
console.log(sum);
return false;
}
</script>
Now that you know the mystery behind a “crazy and stupid Javascript add”, solving it is as easy as parsing the string into a number. There are a number of ways to parse a string into a number:
parseInt(N)
Parse as an integer, drops all decimal places.parseFloat(N)
Parse as float, retains decimal places.Number(N)
Parse as float, retains decimal places.+N
Shorthand, retains all decimal places.
3) ROUNDING ERROR & PRECISION
// (PART A) FLOATING POINT ROUNDING ERROR
let sum = 0.1 + +"0.2";
console.log(sum); // 0.30000000000000004
// (PART B) TO PRECISION
console.log(sum.toPrecision(3)); // 0.300
console.log(sum.toFixed(2)); // 0.30
Here’s one more bit that beginners should be aware of – Javascript is prone to rounding error. Long story short, we use a base 10 decimal system, computers use a base 2 binary system. Javascript adopts IEEE 754 double-precision which sometimes result in errors – Read more on this if you want.
To “solve” this issue on rounding error, you will have to round off the number manually:
N.toPrecision()
To the specified number of significant digits.N.toFixed()
To the specified number of decimal places.
THE END – NUMBER DATA TYPE IN JAVASCRIPT
That’s all for this short tutorial and sharing. One last thing to take note:
console.log(typeof 1)
will give youNumber
.console.log(typeof 1.1)
will also give youNumber
.
Javascript does not distinguish between integer and decimal internally. There is only a Number
data type, which is essentially a float.
CHEAT SHEET
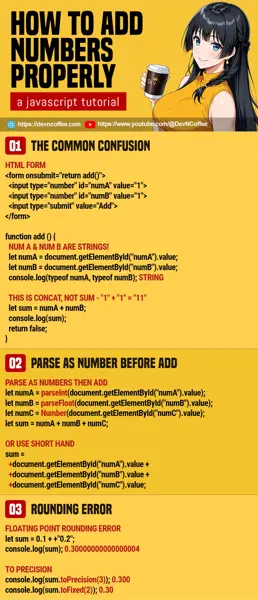