So, you need to append new rows to a CSV file? Prepend rows to the top, or insert in the middle? There’s no need to upload the CSV file to the server, we can directly modify the CSV file in Javascript. Let us walk through a simple example, let’s go!
CODE DOWNLOAD
I have released this under the MIT license, feel free to use it in your own project – Personal or commercial. Some form of credits will be nice though. 🙂
VIDEO TUTORIAL
1) THE HTML
modify-csv.html
<input type="file" accept=".csv" id="demo">
Well, only a file picker to choose a CSV file.
2) THE JAVASCRIPT
modify-csv.js
document.getElementById("demo").onchange = e => {
// (PART A) READ SELECTED FILE
CSV.fetch({
file : e.target.files[0],
noHeaderRow : true
})
// (PART B) MODIFY CSV
.then(data => {
// (B1) APPEND : ADD TO END OF ARRAY
data = data.records;
data.push(["Append", "Row"]);
// (B2) PREPEND : ADD TO START OF ARRAY
data.unshift(["Prepend", "Row"]);
// (B3) INSERT : ADD TO MIDDLE OF ARRAY
data.splice(2, 0, ["Insert", "Row"]);
// (B4) INSERT BEFORE "THIRD"
let at = null;
for (let [i,row] of Object.entries(data)) {
if (row.includes("Third")) { at = i; break; }
}
if (at !== null) {
data.splice(at, 0, ["Before", "Third"]);
}
// (B5) FORCE DOWNLOAD UPDATED CSV
let blob = new Blob([CSV.serialize(data)], {type: "text/csv"}),
url = window.URL.createObjectURL(blob),
a = document.createElement("a");
a.href = url; a.download = "updated.csv";
a.click(); a.remove();
window.URL.revokeObjectURL(url);
});
};
This may be a handful for the beginners, but keep calm and drink coffee. It’s very straightforward:
- To work with the CSV file, we will use this simple CSV library.
- (A) When the user picks a CSV file, we read it using the library.
- (B) Contents of the CSV file will be placed in a
data
array.- (B1) To append to the end, simply
push()
another row to thedata
array. - (B2) To prepend to the start, use
unshift()
to add to the start of thedata
array. - (B3) To insert at a specific row, we use
splice(AT, REMOVE, ROW)
. - (B4) If you do not know the exact row of where to insert – The only way is to loop through the entire array to find the row.
- (B5) Lastly, export the updated
data
array as a CSV file.
- (B1) To append to the end, simply
THE END – A COUPLE OF NOTES
That’s all for this short tutorial and example. Just a couple of notes before we end:
- There is something called “limited system resources” on every device. Although most modern devices are capable of handling large files, I will still recommend putting a limit on it. Do a file size check before using the library to read the chosen CSV file – if (e.target.files[0].size > 987654321) { NOPE } else { PROCEED }
- It is possible to “directly open and save the CSV file without force download” using open file picker. But take note, this is experimental and only available on some browsers at the time of writing.
- Lastly, we can also open a folder, open all CSV files inside. Check out my other tutorial on loading files in Javascript.
CHEAT SHEET
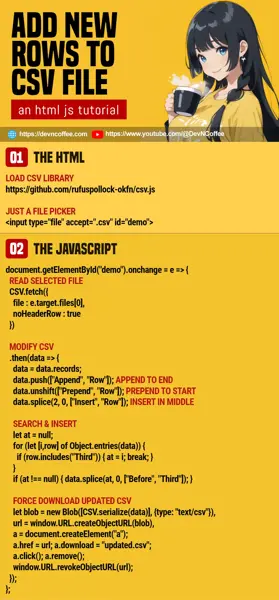