So you need to read a CSV file into an array or object in Javascript? Turn it into a JSON encoded string? Let Master Coffee walk you through some simple examples, let’s go.
CODE DOWNLOAD
I have released this under the MIT license, feel free to use it in your own project – Personal or commercial. Some form of credits will be nice though. 🙂
VIDEO TUTORIAL
DUMMY CSV
Fruit | Color | Taste |
Mango | Yellow | Sweet |
Kiwi | Brown | Tart |
Pineapple | Yellow | Tangy |
Dragonfruit | Pink/Purple | Mildly Sweet |
Persimmon | Orange | Sweet |
For this example, we will work with a simple list of fruits.
1) CSV TO JAVASCRIPT ARRAY
<!-- (PART A) CSV FILE PICKER -->
<input type="file" accept=".csv" id="demoA">
<!-- (PART B) CSV TO ARRAY -->
<script src="csv.min.js"></script>
<script>
document.getElementById("demoA").onchange = e => {
// (B1) READ SELECTED FILE
CSV.fetch({
file : e.target.files[0],
noHeaderRow : true
})
// (B2) TO ARRAY & JSON
.then(data => {
console.log(data.records);
console.log(JSON.stringify(data.records));
});
};
</script>
DATA.RECORDS IS A NESTED ARRAY OF THE CSV FILE:
[
['Fruit', 'Color', 'Taste'],
['Mango', 'Yellow', 'Sweet'],
['Kiwi', 'Brown', 'Tart'],
['Pineapple', 'Yellow', 'Tangy'],
['Dragonfruit', 'Pink/Purple', 'Mildly Sweet'],
['Persimmon', 'Orange', 'Sweet']
]
JSON-ENCODED:
[["Fruit","Color","Taste"],["Mango","Yellow","Sweet"],["Kiwi","Brown","Tart"],["Pineapple","Yellow","Tangy"],["Dragonfruit","Pink/Purple","Mildly Sweet"],["Persimmon","Orange","Sweet"]]
- Start by creating a file picker that only accepts CSV files.
- On choosing a CSV file, read it into an array. While we can manually parse the CSV file, it is easier to just use a CSV library to do it.
- (B1) As according to the CSV library’s documentation, we have to first use
CSV.fetch()
to get the CSV file. In this case, we point it to the selected CSV file in the file picker. - (B1) Take note of the
noHeaderRow : true
setting here. This will also include the first row as data, remove this setting if you do not want the first row. - (B2) On opening the CSV file,
data.records
will contain the data array. If you need it in JSON format, just JSON encode it withJSON.stringify()
.
- (B1) As according to the CSV library’s documentation, we have to first use
2) CSV TO JAVASCRIPT OBJECT
<!-- (PART A) CSV FILE PICKER -->
<input type="file" accept=".csv" id="demoB">
<!-- (PART B) CSV TO OBJECT -->
<script src="csv.min.js"></script>
<script>
document.getElementById("demoB").onchange = e => {
// (B1) READ SELECTED FILE
CSV.fetch({
file : e.target.files[0]
})
// (B2) TO OBJECT & JSON
.then(data => {
let obj = {};
for (let [i, header] of Object.entries(data.fields)) {
obj[header] = [];
for (let row of data.records) { obj[header].push(row[i]); }
}
console.log(obj);
console.log(JSON.stringify(obj));
});
};
</script>
OBJ CONTAINS THE CSV DATA IN THE FORMAT OF:
{
Color : ['Yellow', 'Brown', 'Yellow', 'Pink/Purple', 'Orange'],
Fruit : ['Mango', 'Kiwi', 'Pineapple', 'Dragonfruit', 'Persimmon'],
Taste : ['Sweet', 'Tart', 'Tangy', 'Mildly Sweet', 'Sweet']
}
JSON-ENCODED:
{"Fruit":["Mango","Kiwi","Pineapple","Dragonfruit","Persimmon"],"Color":["Yellow","Brown","Yellow","Pink/Purple","Orange"],"Taste":["Sweet","Tart","Tangy","Mildly Sweet","Sweet"]}
If you want the CSV to be in an object, some “data yoga” is required.
- (A & B) Pretty much the same file picker, and read the CSV file on choosing a file.
- (B2) But take note of how the data is being reshuffled here.
- Create an empty
let obj = {}
. - Loop through the header cells, create an empty array –
obj[HEADER] = []
. - Loop through the data cells, append to the array –
obj[HEADER].push(CELL)
. - Similarly, just do a
JSON.stringify()
if you need it in JSON format.
- Create an empty
Of course, this is only an example. Feel free to reshuffle the object however you wish, maybe obj = [{HEADER: DATA}, {HEADER: DATA}]
.
FETCH CSV FILE ON SERVER
For those who are too lazy to read the documentation, we can also fetch a CSV file on the server – CSV.fetch({ url : "http://site.com/my.csv" }).then(...)
.
THE END – A NOTE ON SYSTEM RESOURCES
That’s all for this short tutorial and sharing, just one small note before we end. Most modern devices are capable and have ample system memory, but there is still something called “limited system resources”.
Most devices can handle large CSV files, but not the massive ones. You may want to limit the file size, stop processing if the selected CSV is too big – if (e.target.files[0].size > 12345678) { alert("File size is too large."); return; }
CHEAT SHEET
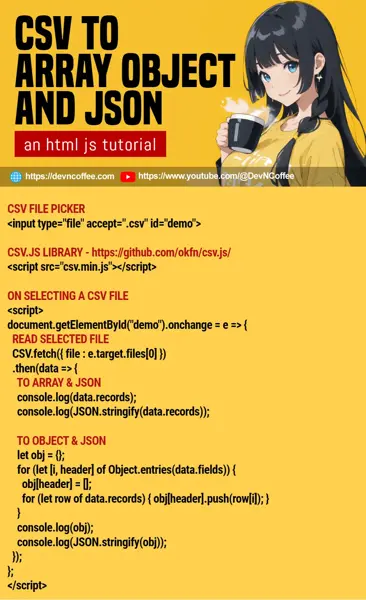