Need to export a generated table in your web app to a CSV file? Yes, it is possible to do that directly in modern Javascript. There’s no need to do the roundabout thing of sending the data to the server and forcing a download again. Let Master Coffee walk you through a simple example – Let’s go!
CODE DOWNLOAD
I have released this under the MIT license, feel free to use it in your own project – Personal or commercial. Some form of credits will be nice though. 🙂
VIDEO TUTORIAL
EXPORT TABLE TO CSV DEMO
Espresso | Strong | Rich, chocolatey, nutty |
Cold Brew | Medium-Strong | Smooth, slightly sweet, chocolaty |
Latte | Weak | Mild coffee aroma with dominant milk |
Cappuccino | Weak | Espresso aroma balanced with steamed milk |
Turkish Coffee | Strong | Rich, intense, slightly smoky |
PART 1) THE HTML
<!-- (PART A) LOAD CSV.JS -->
<script src="csv.min.js"></script>
<script src="table-csv.js"></script>
<!-- (PART B) TABLE TO CONVERT -->
<table id="demo">
<tr>
<td>Espresso</td>
<td>Strong</td>
<td>Rich, chocolatey, nutty</td>
</tr>
<tr>
<td>Cold Brew</td>
<td>Medium-Strong</td>
<td>Smooth, slightly sweet, chocolaty</td>
</tr>
<tr>
<td>Latte</td>
<td>Weak</td>
<td>Mild coffee aroma with dominant milk</td>
</tr>
<tr>
<td>Cappuccino</td>
<td>Weak</td>
<td>Espresso aroma balanced with steamed milk</td>
</tr>
<tr>
<td>Turkish Coffee</td>
<td>Strong</td>
<td>Rich, intense, slightly smoky</td>
</tr>
</table>
<input type="button" value="Download CSV" onclick="tocsv()">
- We can “manually write CSV”, but let’s keep things simple – Use a lightweight csv.js library to do the work.
- Just a “normal HTML table” and a button to start the download.
PART 2) THE JAVASCRIPT
function tocsv () {
// (PART A) TABLE TO ARRAY
let data = [];
for (let tr of document.querySelectorAll("#demo tr")) {
let row = [];
for (let td of tr.querySelectorAll("td")) { row.push(td.innerHTML); }
data.push(row);
}
// (PART B) ARRAY TO BLOB
let blob = new Blob([CSV.serialize(data)], {type: "text/csv"});
// (PART C) DOWNLOAD AS CSV FILE
let url = window.URL.createObjectURL(blob),
a = document.createElement("a");
a.href = url;
a.download = "demo.csv";
a.click();
a.remove();
window.URL.revokeObjectURL(url);
}
To “convert” the table into a CSV file:
- Loop through the table rows and cells. Collect the data in a nested array –
[[CELL, CELL, CELL], [CELL, CELL, CELL], ...]
- Convert the array into a blob (binary object).
- Use the library to turn the array into a “CSV string” –
CSV.serialize(data)
. - Create a blob with the “CSV string”.
- Use the library to turn the array into a “CSV string” –
- Force download the blob as a CSV file.
- Create a URL for the blob –
window.URL.createObjectURL(blob)
- Create an anchor tag.
- Set the blob as the download link of the anchor tag –
<a href="CSV BLOB" download="FILE.CSV">
- Click on the anchor tag to start downloading.
- Clean up – Remove the anchor tag and URL.
- Create a URL for the blob –
THE END – A NOTE ON PERFORMANCE
That’s all for this short and simple tutorial. Before we end, here’s a quick reminder that all systems have limited resources. If you are working with a massive table, you may want to limit the total number of rows or split into multiple CSV files. If you want to do the “opposite” – Check out my other tutorial on displaying a CSV file in an HTML table.
CHEAT SHEET
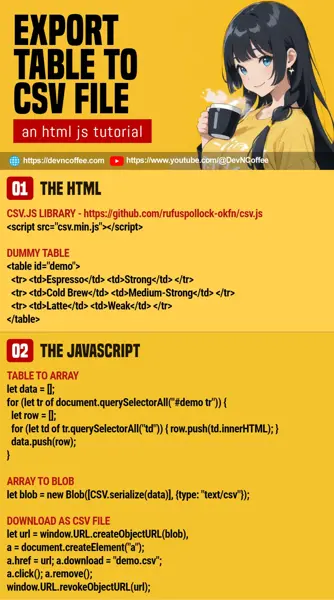