Once upon a time, a student confidently brushed off “round off numbers” in Javascript, because it is “so easy”. Then, she froze in a quiz when asked to “round a number to 3 decimal places” and “round to nearest 5 cents”. Well, let Master Coffee show you how, let’s go.
CODE DOWNLOAD
I have released this under the MIT license, feel free to use it in your own project – Personal or commercial. Some form of credits will be nice though. 🙂
VIDEO TUTORIAL
1) ROUND OFF
// (PART A) ROUND OFF
var demoA = Math.round(0.5);
console.log(demoA); // 1
var demoB = Math.round(0.49);
console.log(demoB); // 0
The “default round off” in Javascript that everyone should be aware of – Math.round()
. How it works is very simple:
- Round up if the first decimal place is more than or equal to
0.5
. - Round down if the first decimal place is less than
0.5
.
2) FLOOR & CEILING
// (PART B) FLOOR & CEILING
var demoA = Math.floor(1.98);
console.log(demoA); // 1
var demoB = Math.ceil(2.34);
console.log(demoB); // 3
Floor and ceiling is the next “Javascript default round off” that everyone should know.
floor()
Drops the decimal value regardless.ceil()
Rounds up to the nearest whole number, regardless of decimal value.
3) ROUND TO NEAREST N DECIMAL PLACE
// (PART C) ROUND OFF TO N DECIMAL PLACES
// (C1) ROUND OFF FUNCTION
var roundto = (num, places) => {
var offset = +("1" + ("0".repeat(places)));
return Math.round(num * offset) / offset;
};
// (C2) ROUND OFF DEMO
var demoA = 1.345;
console.log(roundto(demoA, 2)); // 1.35
var demoB = 2.123456;
console.log(roundto(demoB, 3)); // 2.123
var demoC = 3.3;
console.log(roundto(demoC, 99)); // 3.3
How do we round off to 1, 2, 3, N decimal places? The formula is easy:
- To round off to 1 decimal places –
NUM = Math.round(NUM * 10) / 10
- To round off to 2 decimal places –
NUM = Math.round(NUM * 100) / 100
- To round off to 3 decimal places –
NUM = Math.round(NUM * 1000) / 1000
We can “automate” this by creating a simple function to calculate the “10 offset”:
OFFSET = PARSE INTEGER ("1" + (REPEAT "0" ACCORDING TO NUMBER OF DECIMAL PLACES))
NUM = Math.round(NUM * OFFSET) / OFFSET
4) ROUND TO NEAREST 5 CENT
// (PART D) ROUND OFF TO NEAREST 5 CENT
// (D1) ROUND OFF FUNCTION
var roundcent = amount => {
// (D1-1) SPLIT & CHECK AMOUNT
let split = amount.toString().split(".");
if (split.length!=2 || split[1].length<2) { return amount; }
// (D1-2) NUMBER YOGA
split[0] = +split[0]; // whole number
split[2] = +split[1][1]; // 2nd decimal
split[1] = +split[1][0]; // 1st decimal
// (D1-3) ROUND OFF
// less than 0.03, round down to 0
// between 0.03 and 0.07, round to 0.05
// more than 0.07, round up to 0.1
if (split[2] < 3) { split[2] = 0; }
else if (split[2] >=3 && split[2]<=7) {split[2] = 5; }
else {
split[1]++; split[2] = 0;
if (split[1]==10) {
split[0]++; split[1] = 0;
}
}
// (D1-4) RETURN RESULT
return +`${split[0]}.${split[1]}${split[2]}`;
};
// (D2) ROUND OFF DEMO
console.log(roundcent(1.92)); // 1.9
console.log(roundcent(1.94)); // 1.95
console.log(roundcent(1.97)); // 1.95
console.log(roundcent(1.98)); // 2
If you need “specialized round off”, the only way is to create your own function. Here is a “round off to nearest 5 cent” that I created for a POS.
- (D1-1) Split the given amount into:
split[0]
whole numbers.split[1]
decimal numbers.- If the given amount is a whole number or only has 1 decimal place – Return it as it is.
- (D1-2) Further split the numbers.
split[0]
Whole number.split[1]
First decimal place.split[2]
Second decimal place.
- (D1-3) Round off.
- If
DECIMAL < 0.03
, round down to0
. - If
DECIMAL >= 0.03 && DECIMAL <= 0.07
, round to0.05
. - If
DECIMAL >= 0.08
, round up to0.1
– If the first decimal place is over9
, increment the whole number.
- If
- (D1-4) Return the result,
AMOUNT = PARSE INTEGER (split[0] . split[1] split[2])
THE END – BEWARE OF NUMBER & STRING DATA TYPE
That’s all for this quick tutorial and examples. Just one common beginner pitfall to highlight before end:
- This is a string –
"1" + "1" = "11"
- This is a number –
1 + 1 = 2
- If your calculations of “wrong”, remember to parse the variables to numbers.
CHEAT SHEET
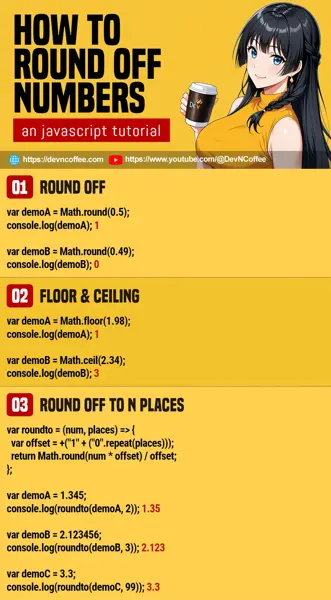